TensorFlow Basic Easy Example in Python
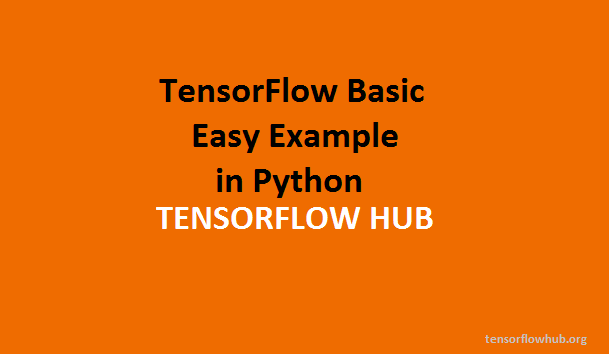
We discussed an easy few lines of code in python in the last tutorial, in this article I will explain the basics of tensorflow functions that we covered in the code, and how it works and how to use some basic operations like the addition, subtraction or multiplication.
Before proceeding I would recommend you to have the tensorflow installed and completely usable as per the installation and setup guide.
You might already be familiar of many commands if you know basics of python, youcan skip them.
To import tensorflow in python
import tensorflow as tf
Tensorflow library has a Variable that can be used easily just like a normal one.
x = tf.Variable([1.0, 2.0])
`tf` is the library that we imported and we create a 1D array with two inputs 1.0and 2.0 both are of float datatype. You can mention datatype and some other attributes separately. I would not go in detail in each of the function as you can find it easily on github and tensorflow official websites.
The following is the simple multiplication command.
z = 2 * x #z will also be a TFVariable
Now comes the tensorflow real stuff. To make use of tensorflow variables and perform calculations using TF, Session must be created and all variables must be initialized using the following statements.
sess = tf.Session() #session is created
init =tf.global_variables_initializer() #initializing variables
Tostart the session;
sess.run(init)
Avoiding this will give issues with the initialization of TF variables.
Now all the variables in the tensorflow graph are ready to be processed. To print any tensorflow value, you will need to run it in a session like this;
print('x = ', sess.run(x))
Since variable is also a TF variable as it is depending on x which is a TF variable. We can get the result like this;
print('z = 2 * x = ',sess.run(z))
Simply printing them would not give the result you expect, it will give you something different, try and let me know what you get.
Here is the complete code, make some changes and play with it for better understanding.
import tensorflow as tf
x= tf.Variable([1.0, 2.0])
z= 2 * xsess= tf.Session()
init= tf.global_variables_initializer()
sess.run(init)
print('x= ', sess.run(x))
print('z= 2 * x = ', sess.run(z))
Incase of any help, feel free to comment and ask anything.